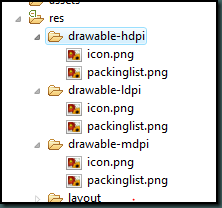

2. Export unsigned application
Right click on the project. Click on “Android Tools->Export Unsigned Application Package…”
2. Generate personal certificate by using keytool in java’s jre\bin directory
3. Sign Android application by using jarsigner in jre\bin direcotry
4. Installation
a) Install application on emulator
b) Install application on device
I downloaded ASTRO File Manager and copied the apk file to device. Use File Manager to find the file and tap on it. It prompted me a couple of questions. I have to change my device setting to allow unknown source apps to be installed on my device.